Python Libraries for Network Engineers
Essential Python libraries that empower network engineers to automate tasks, interact with devices, and analyze network data efficiently.
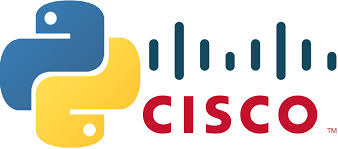
Python Libraries for Network Engineers
Python has become the go-to language for network engineers due to its ease of use and vast ecosystem of libraries designed for network automation, device management, and data analysis. Several Python libraries stand out as essential tools for network engineers, enabling them to automate tasks, interact with network devices, and analyze network data efficiently.
Connection Management & Device Interaction
One of the most widely used libraries is Netmiko, developed by Kirk Byers. Netmiko simplifies SSH connections to network devices and provides an easy way to send commands and retrieve output. It abstracts many complexities of interacting with Cisco, Juniper, Arista, and other vendor devices, making it a preferred choice for automating configuration changes and collecting device data. Another essential library is Paramiko, which provides low-level SSH functionality and is useful when custom SSH interactions are needed beyond what Netmiko offers.
Multi-vendor Automation
For network automation and API-based interactions, NAPALM (Network Automation and Programmability Abstraction Layer with Multivendor support) is a powerful library. It allows engineers to retrieve operational data, manage configurations, and ensure consistency across multiple network vendors using a single API. Similarly, pyATS (Python Automated Test System), developed by Cisco, is widely used for network testing, validation, and verification, helping engineers ensure the reliability of their configurations and deployments.
API & Protocol Libraries
When working with modern network programmability and model-driven automation, libraries like ncclient and requests are invaluable. ncclient is essential for interacting with NETCONF-enabled devices, allowing engineers to retrieve and modify configurations in a structured manner. Requests is widely used for making REST API calls to platforms such as Cisco DNA Center, Cisco Meraki, and Catalyst Center, enabling automation of network management and monitoring tasks.
Analysis & Visualization Tools
In addition to automation libraries, network engineers often rely on Scapy for packet analysis and manipulation. Scapy allows engineers to create, send, and capture packets for network troubleshooting, penetration testing, and protocol development. Furthermore, data analysis libraries like Pandas and Matplotlib are commonly used for processing and visualizing network data, making it easier to identify patterns and optimize network performance.
Empowering Modern Network Operations
By leveraging these Python libraries, network engineers can automate repetitive tasks, enhance network monitoring, and integrate with software-defined networking (SDN) solutions. These tools empower engineers to work more efficiently, reduce manual errors, and keep up with the evolving demands of modern network infrastructures.
# Example: Using Netmiko to configure multiple Cisco devices
from netmiko import ConnectHandler
# Device parameters
devices = [
{
'device_type': 'cisco_ios',
'host': '192.168.1.1',
'username': 'admin',
'password': 'password',
},
{
'device_type': 'cisco_ios',
'host': '192.168.1.2',
'username': 'admin',
'password': 'password',
}
]
# Configuration commands to apply
commands = [
'interface GigabitEthernet0/1',
'description AUTOMATED BY PYTHON',
'ip address 10.10.10.1 255.255.255.0',
'no shutdown'
]
# Connect to each device and apply configuration
for device in devices:
try:
# Establish connection
connection = ConnectHandler(**device)
# Enter configuration mode and apply commands
output = connection.send_config_set(commands)
# Print the output
print(f"Configuration applied to {device['host']}:")
print(output)
# Disconnect from the device
connection.disconnect()
except Exception as e:
print(f"Failed to configure {device['host']}: {str(e)}")